#100DaysOfPython Day 2: Functions, Scope and Best Practices
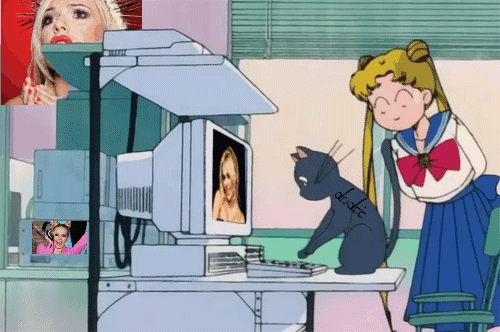
So far I’ve learned a bit about the different types Python posseses and how to print Hello World. Today however, I’ve learned more about Python’s best practices as well as functions and how the language handles access to variables in the local and global scope (spoiler alert: Scope works the same way in Python as it does in JavaScript :-p) .
Update: My collection of notes will always be updated as I learn more things. I’m merely posting as I actively learn. I do not claim to be a Pythonista, I am not an expert.
Advocating for Best Practices
A key quality of an exceptional engineer is consistently writing code that follows best practices. But why is that important? Style guides that contain best practices are basically a set of rules and guidelines that ensures consistency, readability for other humans and efficiency.
The standard that the Python community follows is called PEP-8. My advice, especially when you’re a beginner, is to bookmark this style guide (or the relevant style guide to your programming language/framework) and reference it frequently.
Key tip for early-career developers: The best way to write consistently good code is to always practice and reference standard guides.
Here are just a few of Python’s Best Practices:
Rule | Meaning | Example |
---|---|---|
Function & Variable Names | Be explicit with variable & function names | x = “x is a nondescript variable name” vs favorite_food =” This variable name gives us a better hint at what this piece of data is about” |
Indentation | Indentation is an essential concept in Python | Use 4 spaces per indentation level |
Underscores create more readability | For your descriptive and longer variable and function names, use underscores to make the names more readable | self_care_message() vs. selfcaremessage() |
Refrain from using confusing standalone letters as variable or function names; exception is when they have meaning in math | Using the lowercase letter ‘l’, uppercase letter O or uppercase letter I can be confusing. We don’t write code for ourselves, we write for others. | i = 1 |
All About Functions
Indentation Matters, Ya’ll
Anatomy of a Function:
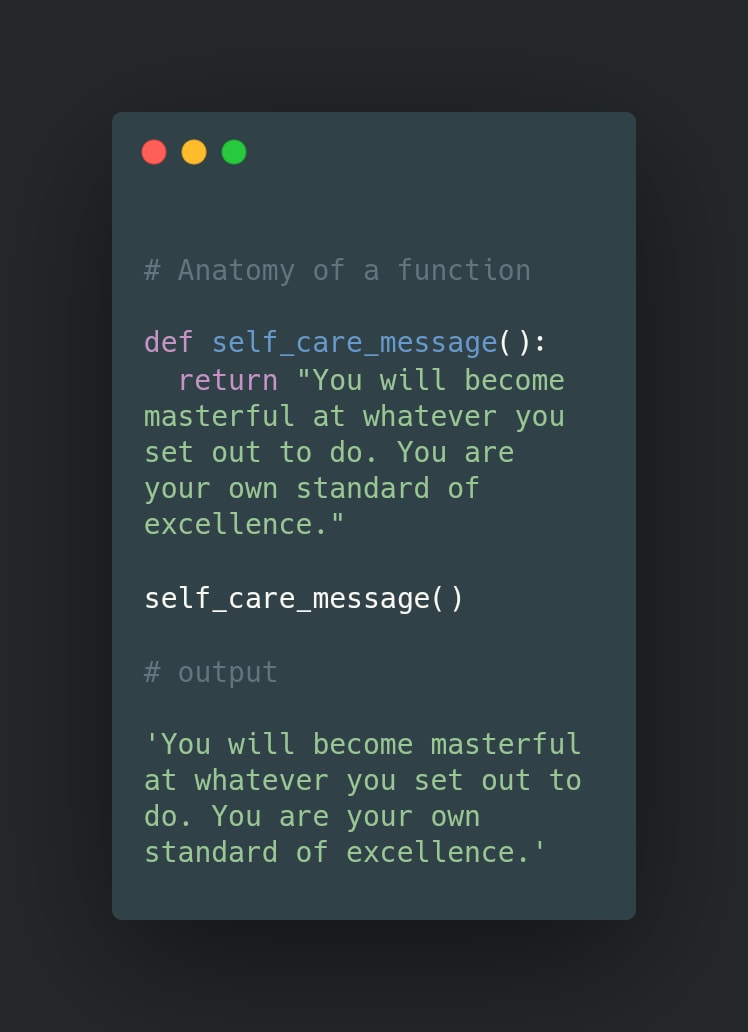
- def is how you initalize the function
- Name the function and be as descriptive as you can be (remember if you’re being verbose with the naming, use underscores in between each word for the function name)
- Follow the function name with parentheses and a colon! (As a Javascript developer I was initially tripped up by this and kept using a semicolon)
- Make an indentation (a tab or 4 spaces) to create your instructions (aka return or print statement)
- Complete and Exit the function by pressing enter
- Call the function by the name you gave it followed by parentheses
Difference between a return statement versus a print statement
A return statement will explicitly return a value.
A print statement will return nothing.

Local and Global Scope
Scope is what determines what variables you can access. I learned in JavaScript that there is a global scope as well as a local scope.
Local scope is when the variables you define within a function can only be accessed in that very function in which you defined it. It won’t be accessible to the global scope (aka rest of the application).
In internal/local scope, the function has access to variables defined within it; has access to variables defined in the global scope (aka rest of application outside that function) but cant change them. Meaning you can’t reassign a global variable within a local function.
Functions without proper whitespace leads to an indentation error.
In production-level code, don’t have too many global variables outside of a defined scope to prevent confusion and bugs; constants are okay to define outside of a scope
Notes on Arguments
Positional arguments are all required and must be given in the order in which they are declared.
Default arguments is kinda like the fallback/default option when you don’t call the last argument when you invoke a function. It is always the last argument in a function.
What Will I Learn Next in Day 3 of the #100DaysOfPython series?
- More advanced data types like sets, tuples, dictionaries