#100DaysOfPython Day 1: Hello World, Data Types & Strings
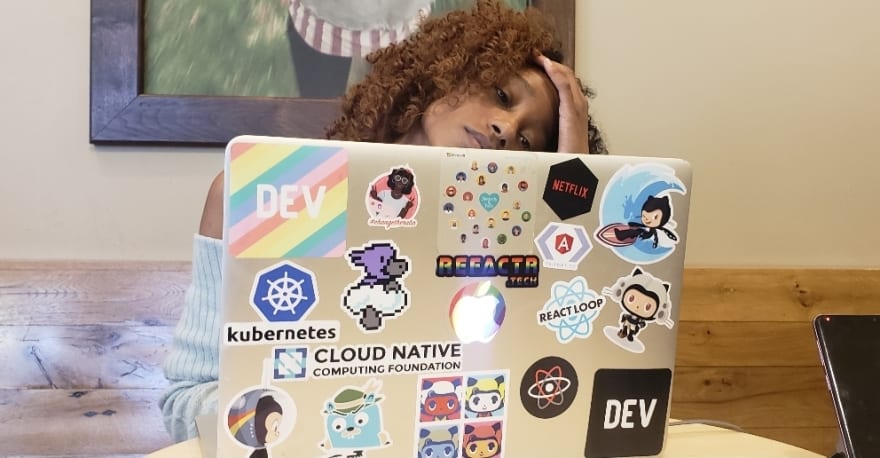
What Led Me To Choose Python As My 2nd Language and why blog?
Frankly, I needed a break from front-end development with JavaScript and wanted to dive deep into a new language. I love how Python is used practically everywhere from machine learning, robotics, fintech, freaking NASA, etc. My goals are to build bots that track the prices of products, create helpful developer tools snd get a better understanding of CS concepts and build full-stack projects. I could’ve easily done all of those things with JS but why not something different?
My mission is to always encourage people to not be afraid to be open about learning something new. We are all a novice at something.
My plan is to make it a nightly habit to convert my handwritten notes into blog posts. I’m treating the series as merely a digital collection of notes to share with the community as opposed to traditional tutorials. I encourage everyone of all experience levels to try as well. It’s a great way to review and solidify your learnings as well as great practice for bettering your communication skills for technical interviews
Update: My collection of notes will always be updated as I learn more things. They are notes, not comprehensive tutorials. I’m merely posting as I actively learn. I do not claim to be a Pythonista, I am not an expert.
The Journey to Hello World

So after installing Anaconda, I started writing Python code using the Jupyter Notebook that’s typically located on localhost:8888.
You use the print() function to print “hello world” and then boom. That’s it.
Data Types
Name | Data Type | Meaning |
---|---|---|
integers | int | whole numbers like 1, 2, 3 |
Floating point | float | numbers with decimal point like 100.00 or 30.2 |
Strings | str | Ordered sequence of characters that uses double or single quotes like “hello” or ‘hello’ |
Lists | list | ordered sequence of objects; similar to JS arrays |
Dictionaries | dict | unordered key:value pairs |
Tuples | tup | ordered immutable sequence of objects |
Sets | set | unordered collection of unique objects |
Booleans | bool | logical value either True or False |
Arithmetic Operations
Operation | Symbol | Meaning | Example |
---|---|---|---|
Addition | + | To Add | 2 + 2 |
Subtraction | – | To Subtract | 2 – 2 |
Division | / | To Divide | 2 / 2 |
Multiplication | * | To Multiply | 2 * 2 |
Modulo Operator | % | To return the remainder after a division | 5 % 5 = 0 |
Variables, Done The Python Way
Python is dynamically typed like JavaScript, meaning you can reassign variables to different data types. Statically typed languages like C++ are more strict because you must define the type of value a variable is set to when you first initialize the variable and prevents the occurrence of bugs.
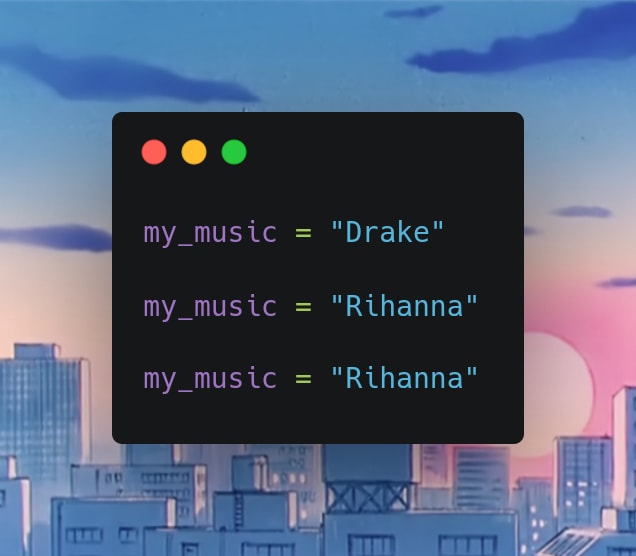
The type() function is a built-in method in Python that lets you check the data type of a variable in case you ever forget!
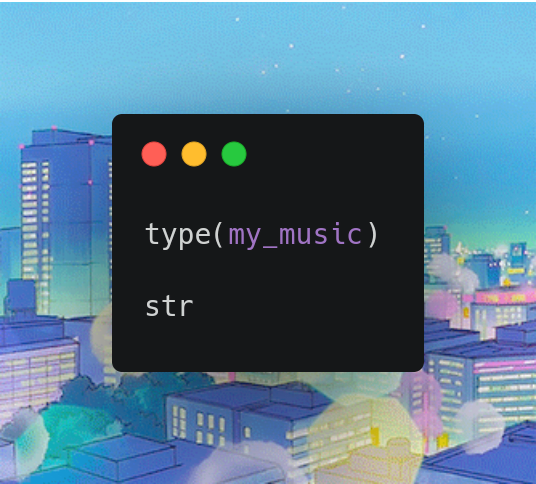
Part I About Strings
Notes about strings will be continued in Day 2 of the #100DaysOfPython series and that will go more in depth about slicing, indexing, print formatting and more which I’m exciting about blogging about tonight because I definitely fucked up on it lol.
A string is an ordered sequences of characters, meaning each character within a string has its own ordered position. This allows you to easily grab or a character within a string using index notation
Character: h e l l o
Index: 0 1 2 3 4
The whitespace within a string count as a character too
Index Notation
Indexing allows you to grab a single character from a string using index notation. Remember space within the string counts as characters!
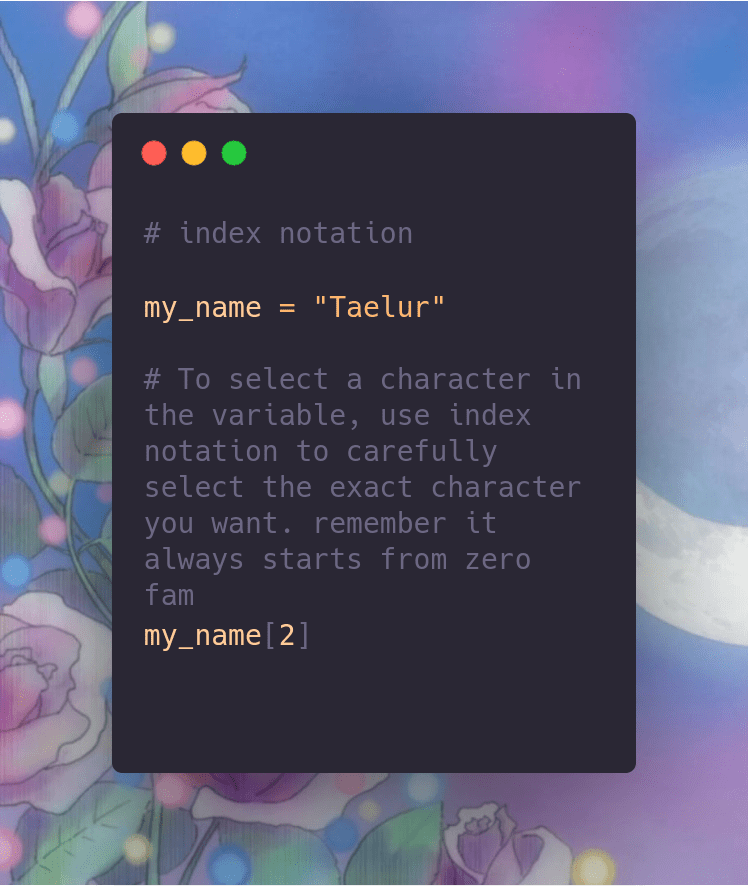
The len function checks the length of a string.
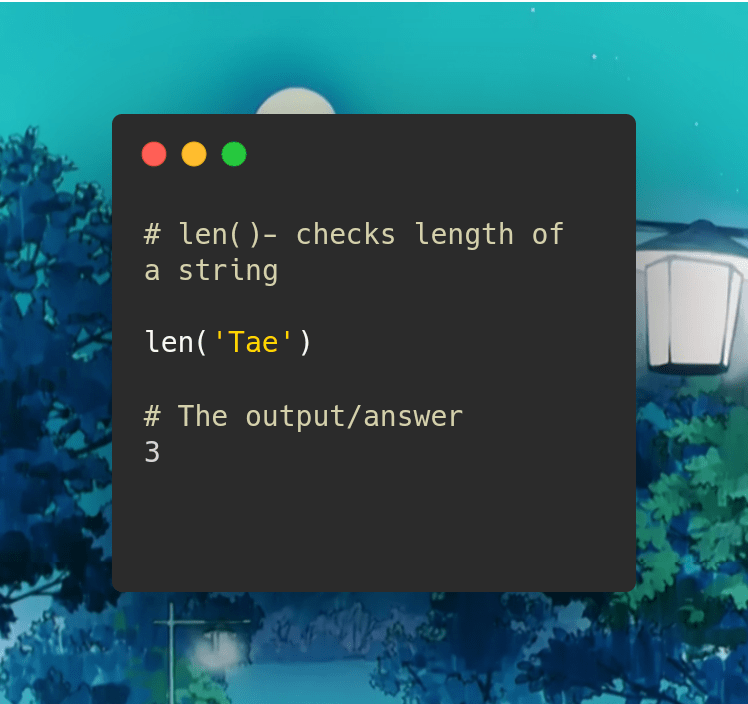
Escape Sequence
Definition: Special commands inside of your string.
Example:
To create a tab in your string use /n
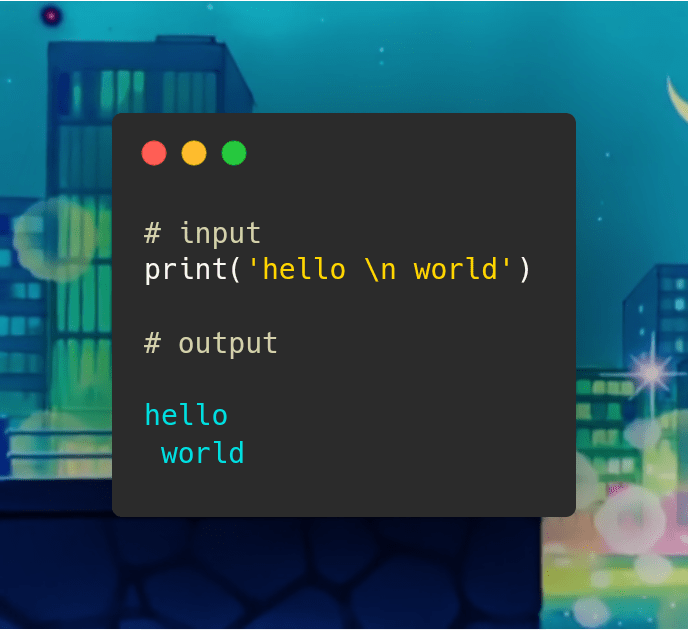
Reverse Indexing in a string
Allows you to grab the last used letter in a string
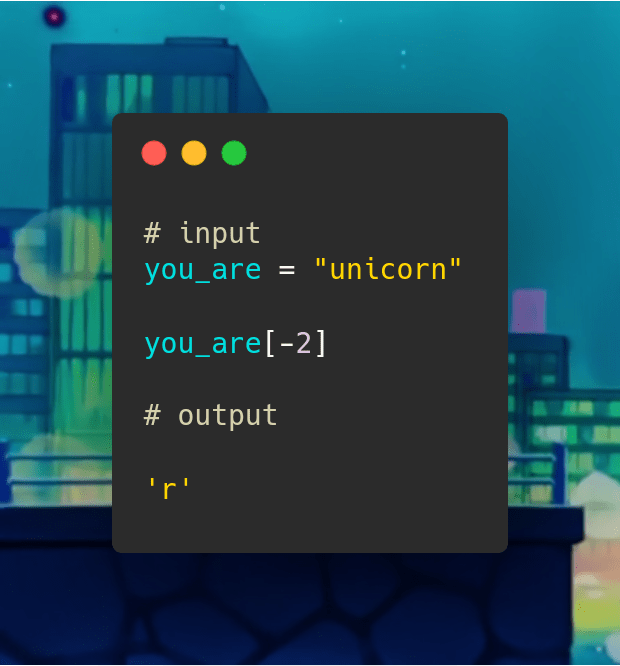
Slicing
Grabs a subset or group of characters in a string
Remember start:stop:step !
Start- A numerical index for starting the slice
Stop- index you will go up to but dont include
Step- size of the leap you take
What Will I Learn Next in Day 2 of the #100DaysOfPython series?
- Lists and Tuples
- Sets and Dictionaries